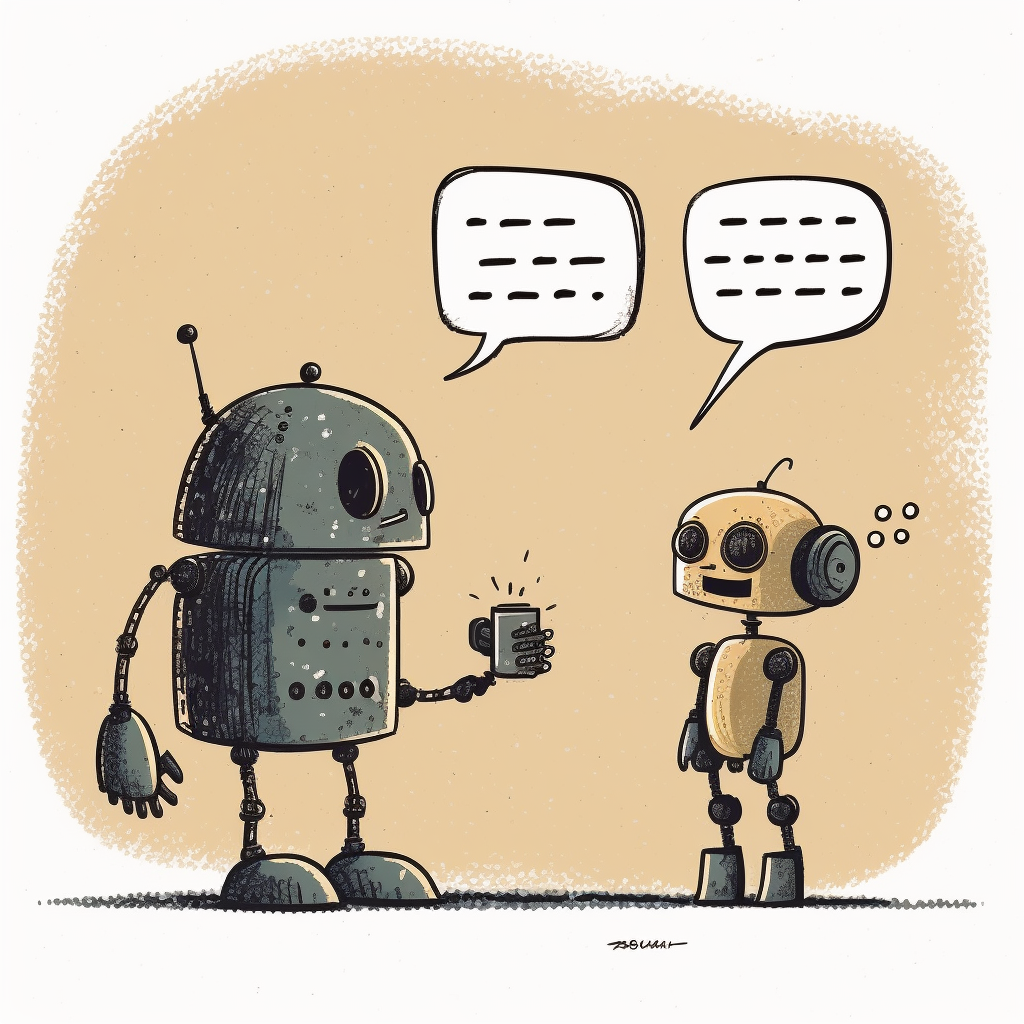
Making Your Python Programs Speak: A Practical Guide to Text-to-Speech | TypeThePipe
In this tutorial, we'll show you how to integrate text-to-speech into your Python projects, using libraries like pyttsx3, gTTS, and playsound. Whether you're building a chatbot, a voice assistant, or just want to add some personality to your applications, this tutorial will help you get started...
