project progress at 100%
0%
100%
- +TODOListManager
- +DrawRnd
- +AXLHub
- +AXLHousing
- +AXNeuroSama
- +AXLNeuroSama
- +AXLearnability
- +TrgTolerance
- +Cycler
- +Perchance
- +AXKeyValuePair
- +InputFilter // context juubi
- +strategy
- +AXStrategy
- +LGTypeConverter
- +OutputDripper
- +PersistantAnswer
- +UniqueItemSizeLimitedPriorityQueue
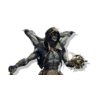
Last edited by a moderator: